需求:创建数据库表与java实体映射,并创建事务保证同时更新或者回滚。
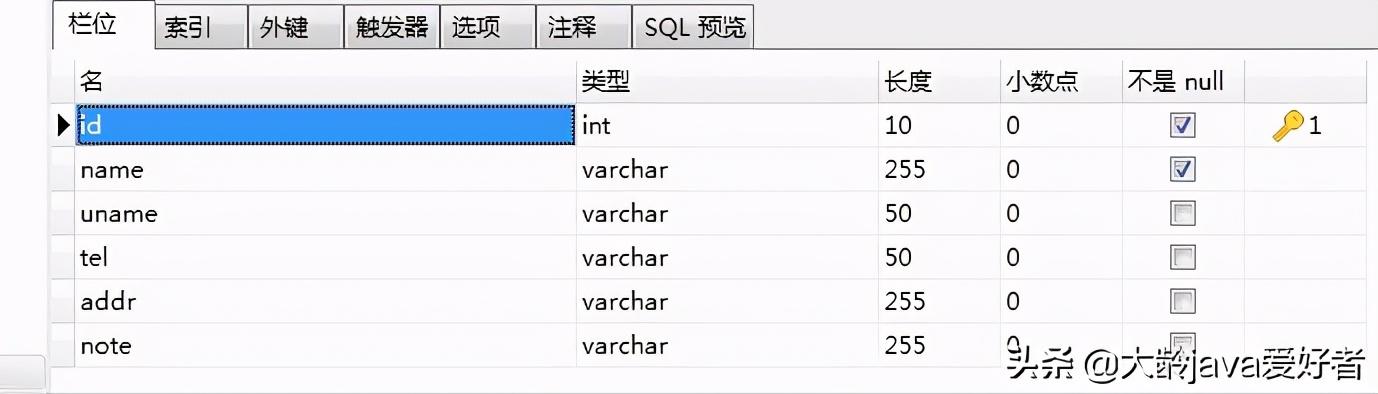
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/test
username=root
password=123456
initialsize=10
minIdle=5
#maxIdle=20 druid不推荐,无效值
maxActive=10
maxWaite=1000
public class Tax {
private int id;
private String name;
private String uname;
private String tel;
private String addr;
private String note;
public Tax() { }
//不需id,数据库id为自缯
public Tax( String name, String uname, String tel, String addr, String note) {
this.name = name;
this.uname = uname;
this.tel = tel;
this.addr = addr;
this.note = note;
}
//getter、setter、toString略
}
public class JdbcUtils {
private static DataSource ds = null;
private static Connection conn = null;
//静态加载配置文件,读取配置创建DataSource对象
static {
Properties p = new Properties();
try {
p.load(JdbcUtils.class.getClassLoader().getResourceAsStream("druid.properties"));
} catch (IOException e) {
e.printStackTrace();
}
try {
ds=DruidDataSourceFactory.createDataSource(p);
} catch (Exception e) {
e.printStackTrace();
}
}
//获取当前连接
public static Connection getConnection() throws SQLException {
//重点,保证当前仅有一个连接
if (conn != null){
return conn;
}
return ds.getConnection();
}
//开启事务,创建当前连接
public static void startTransaction() throws SQLException{
conn = getConnection();
conn.setAutoCommit(false);
}
//提交事务,关闭当前连接
public static void commitTransaction() throws SQLException{
conn.commit();
conn.close();
conn = null;
}
//回滚事务,关闭当前连接
public static void rollBackansaction() throws SQLException{
conn.rollback();
conn.close();
conn = null;
}
}
public class MyDao {
public List<Tax> getAll() throws SQLException {
List<Tax> list = new ArrayList<>();
//获取当前连接
Connection conn = JdbcUtils.getConnection();
Statement statement = conn.createStatement();
ResultSet rs = statement.executeQuery("select * from tax");
while (rs.next()){
Tax tax = new Tax();
tax.setId(rs.getInt("id"));;
tax.setName(rs.getString("name"));
tax.setUname(rs.getString("uname"));
tax.setTel(rs.getString("tel"));
tax.setAddr(rs.getString("addr"));
tax.setNote(rs.getString("note"));
list.add(tax);
}
return list;
}
//数据库插入
public int insertTax(Tax tax) throws SQLException{
//获取当前连接
Connection conn = JdbcUtils.getConnection();
PreparedStatement ps = conn.prepareStatement("insert into tax values (null,?,?,?,?,?)");
ps.setString(1,tax.getName());
ps.setString(2,tax.getUname());
ps.setString(3,tax.getTel());
ps.setString(4,tax.getAddr());
ps.setString(5,tax.getNote());
return ps.executeUpdate();
}
public class TaxService {
public void insertDouble() throws SQLException {
Tax tax1 = new Tax("税务1","张三", "13000000000","","");
Tax tax2 = new Tax("税务2","张三", "13000000000","","");
//注入dao实例
MyDao dao = new MyDao();
try {
//开启事务
JdbcUtils.startTransaction();
dao.insertTax(tax1);
//模拟异常
//int i = 5/0;
dao.insertTax(tax2);
JdbcUtils.commitTransaction();
System.out.println("正常提交");
}catch (Exception e){
JdbcUtils.rollBackansaction();
System.out.println("错误回滚");
}
}
}
public class A {
public static void main(String[] args) throws Exception {
new TaxService().insertDouble();
}
}