
文件上传
- 底层使用的是Apache fileupload组件完成上传,让开发者使用起来更加方便,所以首先需要引入fileupload组件的依赖。
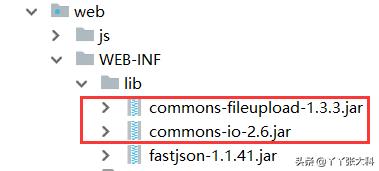
2. 准备 jsp 页面
<%@ page contentType="text/ html ;charset=UTF-8" language=" java " %>
<html>
<head>
<title>上传文件</title>
</head>
<body>
<%--注意:form表单的enctype设置为multipart/form-data,以二进制的形式传输数据。--%>
<form action="upload.do" method="post" enctype="multipart/form-data">
<p>
用户名称:<input type="text" name="userName" />
</p>
<p>
用户密码:<input type="password" name="userPwd" />
</p>
<p>
<%--注意:input的type设置为 file --%>
上传文件:<input type="file" name="userFile"/>
<!-- <input type="file" name="myfile" multiple="multiple"/> multiple多文件-->
</p>
<p>
<input type="submit" value="提交" />
</p>
</form>
</body>
</html>
3.封装上传文件方法工具类
public class FileUploadUtils {
//设置限制总的大小
private static final long TOTALSIZEMAX = 1024*1024*2;
//设置单个文件的大小
private static final long FILESIZEMAX = 1024*1024*1;
//设置允许上传的文件类型
private static final String ALLOWEXT = ".png|.jpg|.gif|.zip|.xlsx";
//设置封装表单对象
public static Map<String,Object> formData;
/**
* 上传文件
* @param request
* @return
*/ public static boolean uploadFile(HttpServlet request request) throws FileUploadException, IOException {
boolean isNoUpload = false;
//第一步:创建上传文件工厂
DiskFileItemFactory diskFileItemFactory = new DiskFileItemFactory();
//第二步:创建上传组件:生成对应的数据参数对象
ServletFileUpload servletFileUpload = new ServletFileUpload(disk File ItemFactory);
//第三步:设置上传文件的字符集
servletFileUpload.setHeaderEncoding("UTF-8");
formData = new HashMap<>();
servletFileUpload.setSizeMax(TOTALSIZEMAX); //设置限制总的大小
servletFileUpload.setFileSizeMax(FILESIZEMAX); //设置限制单个文件的大小
//第四步:生成表单元素的集合
List<FileItem> listItems = servletFileUpload.parseRequest(request);
for (FileItem fileItem : listItems) {
//遍历表单元素的集合
if(fileItem.isFormField()){ //判断是否是表单元素的字段
String formFieldName = fileItem.getFieldName(); //字段的name名称
String formFieldValue = fileItem.getString("UTF-8"); //获取值
formData.put(formFieldName,formFieldValue);
}else{
//文件
//获取上传文件的名称
String uploadName = fileItem.getName(); //判断题.xlsx FSDFSFDSFSDFDSFDSFDS.xlsx
//设置上传文件的路径
String contextPath = request.getServletContext().getRealPath("/");//当前项目的路径
//设置上传路径
String uploadPath = getPath(contextPath); // /upload/2019/08/21/fdsfdsfdsfdsfds.xlsx
//设置限制上传文件的类型
String ext = uploadName.substring(uploadName.lastIndexOf("."),uploadName.length()); //.xlsx
if(ALLOWEXT.indexOf(ext) == -1){ //不能上传该类型
throw new FileUploadException("不支持此格式");
}
//上传文件的名称
String newUploadName = UUIDUtils.getUUId()+ext;
//从表单对象中将文件读取出来
inputStream inputStream = fileItem.getInputStream();
//输出流
FileOutputStream fileOutputStream = new FileOutputStream(contextPath+uploadPath+newUploadName);
byte[] by = new byte[1024];
int len;
while((len = inputStream.read(by)) != -1){
fileOutputStream.write(by,0,len);
}
//关闭输入流
inputStream. close ();
//刷新输出流
fileOutputStream.flush();
fileOutputStream.close();
//存放数据库的文件路径
String saveDB = uploadPath+newUploadName;
formData.put("fileNameUrl",saveDB);
}
}
isNoUpload = true;
return isNoUpload;
}
/**
* 封装上传文件的路径
* @return
*/ public static String getPath(String contextPath){
Calendar calendar = Calendar.getInstance();
//获取年
int year = calendar.get(Calendar.YEAR);
//获取月
int month = calendar.get(Calendar.MONTH)+1;
//获取日
int day = calendar.get(Calendar.DATE);
String uploadPath = File.separator+"upload"+File.separator+year+File.separator+month+File.separator+day+File.separator;
File file = new File(contextPath+uploadPath);
if(!file.exists()){ //判断上传的目录是否存在
//创建
file.mkdirs();
}
return uploadPath;
}
}
- Servlet获取上传文件的表单信息
@WebServlet("/upload.do")
public class UserServlet extends HttpServlet{
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
boolean result = false;
try {
result = FileUploadUtils.uploadFile(request);
if(result){ //上传成功
Map<String,Object> user = FileUploadUtils.formData;
System.out.println(user.get("userName"));
System.out.println(user.get("userPwd"));
System.out.println(user.get("fileNameUrl"));
}
} catch (FileUploadException e) {
e.printStackTrace();
}
}
}
文件下载
- jsp使用超链接,下载准备之前上传fb4b8845778048868c4ae3df9ee2c250.png
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>文件下载</title>
</head>
<body>
<a href="download.do?downloadFileName=fb4b8845778048868c4ae3df9ee2c250.png" style="cursor: pointer">commons-fileupload-1.4-bin.zip</a>
</body>
</html>
2. Servlet中业务方法
异步上传文件
- 准备异步上传jsp页面,注意引入jQuery文件依赖
- 异步上传业务逻辑
需要源码,欢迎留言。如果觉得丫丫分享的还不错,请点击关注。谢谢!
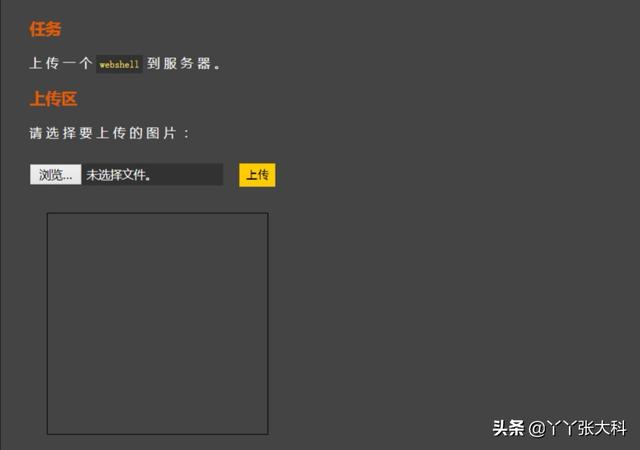