前言
了解JSON的字符串和对象模式以及它们之间的转换;了解AJAX异步的实现和好处;了解I18N如何国际化,即如何更加Locale来切换读取的配置文件。
一、JSON
一种轻量级数据交换格式(与xml做比较),易于阅读和编写,以及程序读取解析,完全独立于不同语言。
1、json的定义
由键值对组成,由花括号包围,键用引号引起,与值用冒号隔开,多组键值对使用逗号隔开。
注:键是字符串,但是值可以有多种类型,有数值型、字符串、布尔型、数组、json,且数组中可以套再套json。
let student = {
“name”: “lls”,
“age”: 18,
“isMarry”: false,
“parentName”: [“root”, “root”],
“parent”: [{
“name”: “root”,
“age”: 50
}, {
“name”: “root”,
“age”: 51,
“isWoman”: false
}]
}
2、json的访问
json为一个普通的对象,key就相当于属性,可以通过打点+属性去获取value。
// json的访问
console.log(student.name)
console.log(student.age)
console.log(student.isMarry)
console.log(student.parent[0].name)
console.log(student.parent[0].age)
console.log(student.parent[1].name)
console.log(student.parent[1].age)
console.log(student.parent[1].isWoman)
3、json的转换
json以对象或者字符串的形式存在,两者之间可以相互转换。
操作数据时,需要json的对象模式;而数据交换时需要json的字符串模式。
// json对象转字符串
console.log(student)
let studentStr = JSON.stringify(student)
console.log(studentStr)
// json字符串转json对象
let studentJson = JSON.parse(studentStr)
console.log(studentJson)
4、json在Java中使用
java中json的常见转换目标,JavaBean、List、Map。需要相关解析json的jar包,这里使用Google的gson.jar。
A.JavaBean
/**
* JavaBean转Json
*/
@Test
public void test1() {
Person p = new Person(“root”, 18, false, new String[]{“root”, “root”},
new Person[]{new Person(“root”, 50, true, null, null),
new Person(“root”, 51, true, null, null)});
//创建Gson对象
Gson gson = new Gson();
//将JavaBean转换为json的字符串模式。
String s = gson.toJson(p);
System.out.println(s);
//输出
//{“name”:”root”,”age”:18,”isMarry”:false,”parentName”:[“root”,”root”],
// “parent”:[{“name”:”root”,”age”:50,”isMarry”:true},{“name”:”root”,”age”:50,”isMarry”:true}]}
//将json的字符串模式转换为JavaBean
Person person = gson.fromJson(s, Person.class);
System.out.println(person);
//输出
//Person{name=’root’, age=18, isMarry=false, parentName=[root, root],
// parent=[Person{name=’root’, age=50, isMarry=true, parentName=[null, null], parent=[null, null]},
// Person{name=’root’, age=51, isMarry=true, parentName=[null, null], parent=[null, null]}]}
}
B.List
/**
* Json 与 List
*/
@Test
public void test2() {
Person p1 = new Person(“root1”, 18, false, null, null);
Person p2 = new Person(“root2”, 19, false, null, null);
List<Person> pList = new ArrayList<>();
pList.add(p1);
pList.add(p2);
//创建Gson对象
Gson gson = new Gson();
//将list转换为json字符串模式
String s = gson.toJson(pList);
System.out.println(s);
//输出
//[{“name”:”root1″,”age”:18,”isMarry”:false},{“name”:”root2″,”age”:19,”isMarry”:false}]
//将json字符串模式转换为List类型
Type type = new PersonListType().getType();
List<Person> personList = (List<Person>)gson.fromJson(s, type);
personList.forEach(System.out::println);
}
package com.xhu.pojo;
import com.google.gson.reflect.TypeToken;
import java.util.List;
public class PersonListType extends TypeToken<List<Person>> {
}
C.Map
/**
* Map 与json的转换
*/
@Test
public void test3() {
Map<Integer, Person> m = new HashMap<>();
for (int i = 0; i < 10; i++) {
Person person = new Person();
person.setName(String.valueOf(i));
m.put(i, person);
}
//创建Gson对象
Gson gson = new Gson();
//Map 转json的字符串模式
String s = gson.toJson(m);
System.out.println(s);
//json字符串模式转Map
Map<Integer, Person> map = gson.fromJson(s, new TypeToken<Map<Integer, Person>>() {
}.getType());
map.values().forEach(System.out::println);
}
二、AJAX
AJAX(Asynchronous JavaScript And XML),XML是一种传输数据的一个格式,现在普遍用JSON。
作用)用js异步发送请求,局部更新数据。
<script type=”text/javascript”>
function ajaxRequest() {
// 1、我们首先要创建XMLHttpRequest
let xhr = new XMLHttpRequest()
// 2、调用open方法设置请求参数,true表示异步,false表示必须同步执行下面的代码,即必须响应回来了才能执行下面的代码。
xhr.open(“get”, “#34;, true);
// 3、在send方法前绑定onreadystatechange事件,处理请求完成后的操作。
xhr.onreadystatechange = function () {
if (xhr.readyState == 4 && xhr.status == 200) {
let object = JSON.parse(xhr.responseText)
console.log(object);
document.getElementById(“#name”).value = object.name
document.getElementById(“#password”).value = object.password
}
}
// 4、调用send方法发送请求
xhr.send()
}
</script>
注:可以参考官方API文档,做到知其然,还知其所以然。
注:也可以参考Jquery的get、post、getJson。
JQuery 中的 Ajax 请求,
$.ajax 方法 url type data success dataType
$.get 方法和$.post 方法 url data callback type
$.getJSON 方法 url data callback
表单序列化 serialize(),将form表单中的name和value转换成name1=value1&name2=value2&…name=value的形式。
三、I18N
1、Core
可选择网页的展现语言,不改变样式,只改变内容。
Core在于,工具类ResourceBundle来关联当地信息Locale和不同语言配置文件,然后根据不同的Locale信息读相应的配置文件信息。
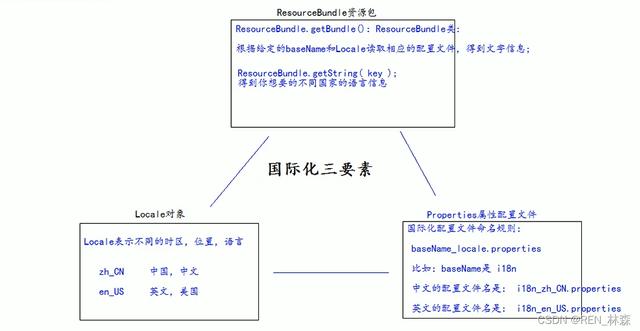
2、Properties
i18n_en_US_properties,
username=username
password=password
sex=sex
age=age
regist=regist
boy=boy
email=email
girl=girl
reset=reset
submit=submit
i18n_zh_CN.properties,
username=用户名
password=密码
sex=性别
age=年龄
regist=注册
boy=男
girl=女
email=邮箱
reset=重置
submit=提交
3、Java实现
@Test
public void testI18n(){
// 得到我们需要的 Locale 对象
Locale locale = Locale.CHINA;
// 通过指定的 basename 和 Locale 对象,读取 相应的配置文件
ResourceBundle bundle = ResourceBundle.getBundle(“i18n”, locale);
System.out.println(“username:” + bundle.getString(“username”));
System.out.println(“password:” + bundle.getString(“password”));
System.out.println(“Sex:” + bundle.getString(“sex”));
System.out.println(“age:” + bundle.getString(“age”));
}
}
4、JSTL的fmt替代Java的配置和输出
<%–1 使用标签设置 Locale 信息–%>
<fmt:setLocale value=”” />
<%–2 使用标签设置 baseName–%>
<fmt:setBundle basename=””/>
<%–3 输出指定 key 的国际化信息–%>
<fmt:message key=”” />
5、Case
<%@ taglib prefix=”fmt” uri=”#34; %>
<%@ page language=”java” contentType=”text/html; charset=UTF-8″ pageEncoding=”UTF-8″ %>
<!DOCTYPE html PUBLIC “-//W3C//DTD HTML 4.01 Transitional//EN” “#34;>
<html>
<head>
<meta http-equiv=”pragma” content=”no-cache”/>
<meta http-equiv=”cache-control” content=”no-cache”/>
<meta http-equiv=”Expires” content=”0″/>
<meta http-equiv=”Content-Type” content=”text/html; charset=UTF-8″>
<title>Insert title here</title>
</head>
<body> <%–1 使用标签设置 Locale 信息–%>
<fmt:setLocale value=”${param.locale}”/>
<%–2 使用标签设置 baseName–%>
<fmt:setBundle basename=”i18n”/>
<a href=”i18n_fmt.jsp?locale=zh_CN”>中文</a>| <a href=”i18n_fmt.jsp?locale=en_US”>english</a>
<center>
<h1><fmt:message key=”regist”/></h1>
<table>
<form>
<tr>
<td><fmt:message key=”username”/></td>
<td><input name=”username” type=”text”/></td>
</tr>
<tr>
<td><fmt:message key=”password”/></td>
<td><input type=”password”/></td>
</tr>
<tr>
<td><fmt:message key=”sex”/></td>
<td><input type=”radio”/><fmt:message key=”boy”/>
<input type=”radio”/><fmt:message key=”girl”/>
</td>
</tr>
<tr>
<td><fmt:message key=”email”/></td>
<td><input type=”text”/></td>
</tr>
<tr>
<td colspan=”2″ align=”center”>
<input type=”reset” value=”<fmt:message key=”reset” />”/>
<input type=”submit” value=”<fmt:message key=”submit” />”/></td>
</tr>
</form>
</table>
<br/> <br/> <br/> <br/></center>
</body>
</html>
总结
1)JSON
2)AJAX
3)I18N