图片
定义Image :
Image image = new Image(URL);
其中,URL可以指向网络图片、资源图片或本地图片。示例:
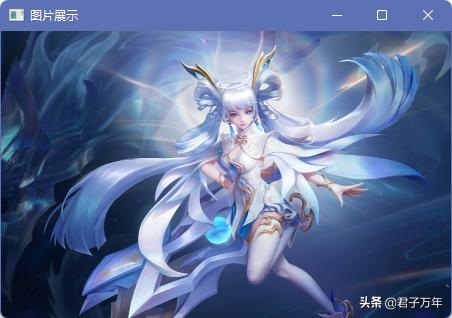
网络图片
网络图片 是指存储在服务器上,可通过网络访问的图片资源。网络图片通常遵循 http 或 https 协议。例如:
Image image = new Image("#34;);
本地图片
本地图片 是指存储在本地的图片资源。本地图片需遵循 File 协议。例如:
Image image = new Image("file:C://SunShangXiang.jpg");
可改写为:
File file = new File("C://SunShangXiang.jpg");
String uri = file.toURI().toString();
Image image = new Image(uri);
资源图片
URL url = ImageApplication.class.getResource("/images/logo.png");
Image image = new Image(url.toURI().toString());
完整代码
package io.weijunfu.javafx;
import javafx .application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import java .io.File;
import java.net.URL;
public class ImageApplication extends Application {
@ Override
public void start(Stage stage) throws Exception {
BorderPane pane = new BorderPane();
// image 用于加载图片
// 来自网络图片
// Image image = new Image("#34;);
// 来自本地图片
// Image image = new Image("file:C://SunShangXiang.jpg");
// File file = new File("C://SunShangXiang.jpg");
// String uri = file.toURI().toString();
// Image image = new Image(uri);
// 来自资源文件
URL url = ImageApplication.class.getResource("/images/logo.png");
Image image = new Image(url.toURI().toString());
double width = image.getWidth();
double height = image.getHeight();
// imageView 用于显示图片
ImageView imageView = new ImageView();
imageView.setImage(image);
pane.setCenter(imageView);
// 创建场景
Scene scene = new Scene(pane, width, height);
// 给舞台添加场景
stage.setScene(scene);
// 设置窗口标题
stage.setTitle("图片展示");
// 显示舞台
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
电子相册

获取图片存储目录
URL url = AlbumApplication.class.getResource("/images/album"); // 图片存储目录
File file = new File(url.toURI());
if(file. exists () && file.isDirectory()) {
files = file.listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
int index = name.lastIndexOf(".");
String suffix = name.substring(index).toLowerCase(Locale.ROOT);
switch (suffix) { // 仅获取jpg和png格式的图片
case ".jpg":
case ".png":
return true;
}
return false;
}
});
if(files.length > 0) { // 默认显示第一张照片
Image image = new Image(files[ current Index].getAbsolutePath());
imageView.setImage(image);
}
}
上一张 & 下一张
// 上一张
private void prevPhone() {
currentIndex--;
currentIndex = currentIndex < 0 ? files.length-1 : currentIndex;
imageView.setImage(new Image(files[currentIndex].getAbsolutePath()));
}
// 下一张
private void nextPhone() {
currentIndex++;
currentIndex = currentIndex >= files.length ? 0 : currentIndex;
imageView.setImage(new Image(files[currentIndex].getAbsolutePath()));
}
完整代码
package io.weijunfu.javafx;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
import java.io.File;
import java.io.FilenameFilter;
import java.net.URL;
import java.util.Arrays;
import java.util. Locale ;
/**
* 电子相册
*
* @Author: weijunfu<ijunfu@163.com>
* @Date: 2021-11-11 22:29
* @Version: 1.0.0
*/
public class AlbumApplication extends Application {
private final static double width = 320;
private final static double height = 240;
private Integer currentIndex = 0;
private File[] files;
private ImageView imageView = new ImageView();
@Override
public void start(Stage stage) throws Exception {
AnchorPane pane = new AnchorPane();
// ImageView imageView = new ImageView();
imageView.setFitWidth(width);
imageView.setFitHeight(height);
imageView.setX(0);
imageView.setY(0);
pane.getChildren().add(imageView);
URL url = AlbumApplication.class.getResource("/images/album"); // 图片存储目录
File file = new File(url.toURI());
if(file.exists() && file.isDirectory()) {
files = file.listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
int index = name.lastIndexOf(".");
String suffix = name.substring(index).toLowerCase(Locale.ROOT);
switch (suffix) { // 仅获取jpg和png格式的图片
case ".jpg":
case ".png":
return true;
}
return false;
}
});
if(files.length > 0) { // 默认显示第一张照片
Image image = new Image(files[currentIndex].getAbsolutePath());
imageView.setImage(image);
}
}
Label leftLabel = new Label("<");
leftLabel.setLayoutX(0);
leftLabel.setLayoutY(0);
leftLabel.setPrefWidth(50);
leftLabel.setPrefHeight(height);
leftLabel.setStyle("-fx-background-color: purple; ");
leftLabel.setOpacity(0.2);
leftLabel.setOnMouseClicked(keyEvent -> {
prevPhone();
});
// pane.getChildren().add(leftLabel);
Label rightLabel = new Label(">");
rightLabel.setLayoutX(width-50);
rightLabel.setLayoutY(0);
rightLabel.setPrefWidth(50);
rightLabel.setPrefHeight(height);
rightLabel.setStyle("-fx-background-color: purple; ");
rightLabel.setOpacity(0.2);
rightLabel.setOnMouseClicked(keyEvent -> {
nextPhone();
});
// pane.setRight(rightLabel);
pane.getChildren().addAll(leftLabel, rightLabel);
Scene scene = new Scene(pane, width, height);
stage.setScene(scene);
stage.setTitle("电子相册");
stage.show();
}
/**
* 上一张照片
* @Author: weijunfu<ijunfu@163.com>
* @Version: 1.0.0
* @Date: 2021/11/12 1:03
* @param
* @Return: void
*/
private void prevPhone() {
currentIndex--;
currentIndex = currentIndex < 0 ? files.length-1 : currentIndex;
imageView.setImage(new Image(files[currentIndex].getAbsolutePath()));
}
/**
* 下一张照片
* @Author: weijunfu<ijunfu@163.com>
* @Version: 1.0.0
* @Date: 2021/11/12 1:02
* @param
* @Return: void
*/
private void nextPhone() {
currentIndex++;
currentIndex = currentIndex >= files.length ? 0 : currentIndex;
imageView.setImage(new Image(files[currentIndex].getAbsolutePath()));
}
public static void main(String[] args) {
launch(args);
}
}