虽然现在短信验证已经最流行也是最常用的验证方式;但是邮件验证还是必不可少,依然是网站的必备功能之一。什么注册验证,忘记密码或者是给用户发送营销信息都是可以使用邮件发送功能的。最早期使用JavaMail的相关api来进行发送邮件的功能开发,后来spring整合了JavaMail的相关api推出了JavaMailSender更加简化了邮件发送的代码编写,现在springboot对此进行了封装就有了现在的spring-boot-starter-mail。
1、新建项目sc-mail,对应的pom.xml文件如下
<project xmlns="" xmlns:xsi="" xsi:schemaLocation=" "> <modelVersion>4.0.0</modelVersion> <groupId>spring-cloud</groupId> <artifactId>sc-mail</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>sc-mail</name> <url> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.4. RELEASE </version> </parent> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Finchley.RELEASE</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <properties> <project. build .sourceEncoding>UTF-8</project.build.sourceEncoding> < maven .compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> </project>
2、新建配置文件application.yml
spring: application: name: sc-mail mail: host: smtp.qq.com #邮箱服务器地址 port: 465 username: 515768476@qq.com #用户名 password: vfcqhwsnnwugbhcx #密码 (改成自己的密码) default-encoding: UTF-8 properties: mail: smtp: ssl: enable: true
3、新建邮件发送服务类
package sc.mail. service .impl; import java.io.File; import javax.mail.internet.Mime message ; import org. slf4j .Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.factory. annotation .Autowired; import org.springframework.core.io.FileSystemResource; import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSender; import org.springframework.mail.javamail.MimeMessageHelper; import org.springframework.stereotype.Service; import sc.mail.service.MailService; @Service public class MailServiceImpl implements MailService { private final Logger logger = LoggerFactory.getLogger(this.getClass()); @Autowired private JavaMailSender mailSender; /** * 文本 * @param from * @param to * @param subject * @param content */ @Override public void sendSimpleMail(String from, String to, String subject, String content) { SimpleMailMessage message = new SimpleMailMessage(); message.setFrom(from); message.setTo(to); message.setSubject(subject); message.setText(content); try { mailSender.send(message); logger.info("simple mail had send。"); } catch (Exception e) { logger.error("send mail error", e); } } /** * @param from * @param to * @param subject * @param content */ public void sendTemplateMail(String from, String to, String subject, String content) { MimeMessage message = mailSender.createMimeMessage(); try { //true表示需要创建一个multipart message MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); helper.setText(content, true); mailSender.send(message); logger.info("send template success"); } catch (Exception e) { logger.error("send template eror", e); } } /** * 附件 * * @param from * @param to * @param subject * @param content * @param filePath */ public void sendAttachmentsMail(String from, String to, String subject, String content, String filePath){ MimeMessage message = mailSender.createMimeMessage(); try { MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); helper.setText(content, true); FileSystemResource file = new FileSystemResource(new File(filePath)); String fileName = filePath.substring(filePath.lastIndexOf(File.separator)); helper.addAttachment(fileName, file); mailSender.send(message); logger.info("send mail with attach success。"); } catch (Exception e) { logger.error("send mail with attach success", e); } } /** * 发送内嵌图片 * * @param from * @param to * @param subject * @param content * @param imgPath * @param imgId */ public void sendInlineResourceMail(String from, String to, String subject, String content, String imgPath, String imgId){ MimeMessage message = mailSender.createMimeMessage(); try { MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(from); helper.setTo(to); helper.setSubject(subject); helper.setText(content, true); FileSystemResource res = new FileSystemResource(new File(imgPath)); helper.addInline(imgId, res); mailSender.send(message); logger.info("send inner resources success。"); } catch (Exception e) { logger.error("send inner resources fail", e); } } }
4、新建测试类
package sc.mail; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; import sc.mail.service.MailService; @RunWith(SpringRunner.class) @SpringBootTest public class MailSendTest { @Autowired private MailService mailService; @Test public void sendSimpleMailTest() { mailService.sendSimpleMail("515768476@qq.com", "happy.huangjinjin@163.com", "sendSimpleMailTest", "sendSimpleMailTest from 515768476@qq.com"); } @Test public void sendTemplateMailTest() { String html = "<html><body>" + " <div> " + " sendTemplateMailTest from 515768476@qq.com </br>" + " <b>这是模板邮件</b>" + "</div>" + "</body></html>"; mailService.sendTemplateMail("515768476@qq.com", "happy.huangjinjin@163.com", "sendTemplateMailTest", html); } @Test public void sendAttachmentsMailTest() { String filePath = "D:\springcloudws\sc-mail\src\main\java\sc\mail\service\impl\MailServiceImpl.java"; mailService.sendAttachmentsMail("515768476@qq.com", "happy.huangjinjin@163.com", "sendAttachmentsMailTest", "sendAttachmentsMailTest from 515768476@qq.com", filePath); } @Test public void sendInlineResourceMailTest() { String imgId = "img1"; String content = "<html><body>" + "sendInlineResourceMailTest:<img src='cid:" + imgId + "' >" + "</body></html>"; String imgPath = "D:\springcloudws\sc-mail\src\main\resources\20181015223228.jpg"; mailService.sendInlineResourceMail("515768476@qq.com", "happy.huangjinjin@163.com", "sendAttachmentsMailTest", content, imgPath, imgId); } }
5、运行测试类验证是否发送邮件成功
登录happy.huangjinjin@163.com邮箱
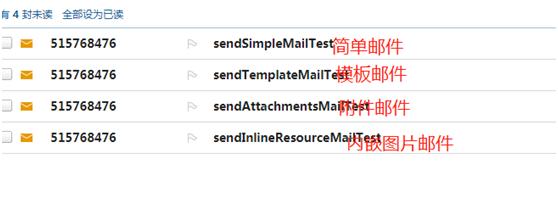
简单邮件
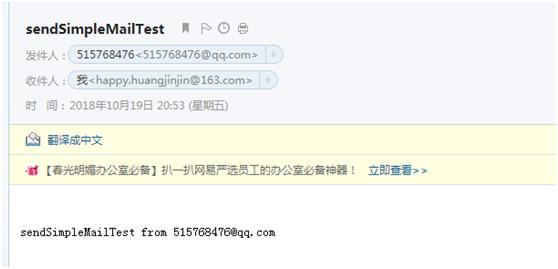
模板邮件
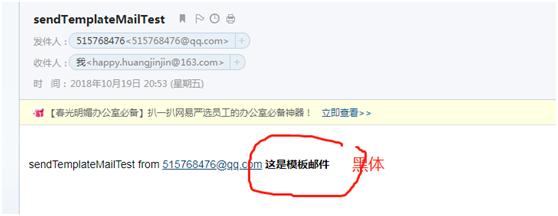
附件邮件

内嵌图片邮件
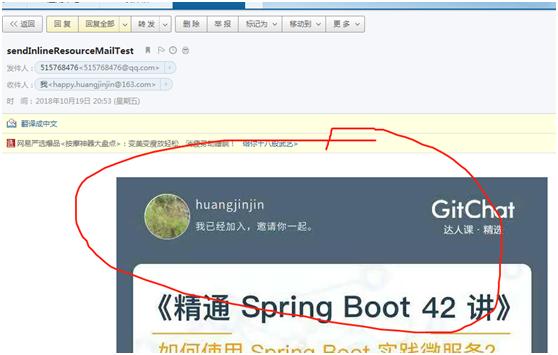