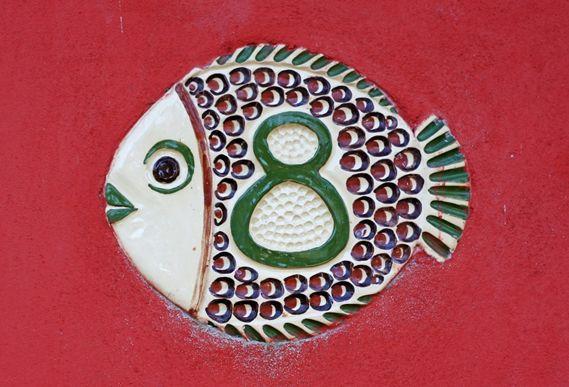
海中有大量的注解!
java SE 1.5中首次引入了注解。Java注解的目的是允许程序员编写关于其程序的元数据。在OracleDocs中,注解的定义是:“注解是元数据的一种形式,它提供的数据与程序本身无关。”
注解可以在代码的任何地方使用,如在类、方法和变量中使用。从Java 8开始,它也可以用于类型声明。
带注解的代码与程序没有任何直接关系。只有其他程序或 JVM 可以使用这些信息来实现其目的。
注解的语法
注解是使用字符@和注解名(即@ annotation Name)来声明的。当编译器遍历这个元素时,即可知道这是一个注解。例如:
@ExampleAnnotation
publicclass SampleClass {
}
上面的注解称为ExampleAnnotation,用于注解类SampleClass。
注解可以有许多属性。这些属性会在声明注解时以键-值对的形式给出。如:
@ExampleAnnotation(name = ”first name”, age = 35)
publicvoid simpleMethod() {
}
注意,这里的ExampleAnnotation是在注解一个方法。如果注解只有一个属性,那么在声明注解时可以跳过该属性的名称。举例如下:
@ExampleAnnotation(“I am the only property”)
publicvoid simpleMethod() {
}
一个元素可以有多个注解。例如:
@Annotation1
@Annotation2(“Another Annotation”)
publicclass SimpleClass {
}
表J2SE 8;相同的注解可以多次用于一个元素,如:
@ExampleAnnotation(“Annotation used”)
@ExampleAnnotation(“Annotation repeated”)
publicclass SimpleClass {
}
这将在@Repeatable注解部分进行详细讨论。
Java中预定义的注解
Java附带了一组预定义的注解。Java Core提供的注解如下:
@Retention:这个注解注解其他注解,并指示注解的范围。可能的值包括:
- SOURCE — 表示该注解只在源代码中可用,编译器和JVM会忽略它,因此在运行时不可用。
- CLASS — 表示此注解对编译器可用,但对JVM不可用,因此在运行时不可用。
- RUNTIME —表示该注解对JVM可用,因此可以在运行时使用。
@Target: 该注解表示一个注解可以应用到的目标元素:
- Annotation _TYPE — 意味着可将该注解应用于其他注解。CONSTRUCTOR — 可以应用于构造函数。
- FIELD — 可以应用于字段或属性。
- LOCAL_VARIABLE —可应用于局部字段。
- METHOD— 可用于方法。
- PACKAGE— 可用于程序包声明。
- PARAMETER — 可用于方法的参数。
- TYPE — 可用于Class, Interface, Annotation, 或列举声明.
- PACKAGE— 可应用于程序包声明。
- TYPE_PARAMETER — 可应用于类型参数声明。
- TYPE_USE — 可应用于任何类型。
@Documented:此注解可应用于其他注解。这意味着将使用Javadoc工具记录带注解的元素。
@Inherited:默认情况下,注解不会被子类继承。但是如果一个注解被标记为@ inherated,这意味着当一个类被注解时,这个注解也会被子类继承。此注解仅适用子类。注意,如果一个接口是用那个注解注解的,那么实现类不会继承这个注解。
@Deprecated:指示不应使用带注解的元素。此注解使编译器生成警告消息,可以应用于方法、类和字段。
@SuppressWarnings:指示编译器出于某种或某些特定原因不生成警告消息。
@Override: 该注解通知编译器该元素正在重写超类的一个元素。在重写元素时不强制使用,但有助于编译器在重写未正确完成时生成出错消息,例如,如果子类方法参数与超类参数不同,或者返回类型不匹配。
@SafeVarargs: 当应用到一个方法或构造方法时,代码不会对可变参数执行潜在的不安全操作。
重复注解
此注解表明,使用此注解的注解可以多次应用于同一元素。
通过一个例子可以更清楚地理解这个概念。
若要使用这个注解,首先,我们需要定义一个注解,它可用于对类进行重复注解。
@Retention (RetentionPolicy.RUNTIME)
@Target (ElementType.TYPE_USE)
@Repeatable (RepeatableAnnotationContainer.class)
public@interface RepeatableAnnotation() {
String values();
}
此处,RepeatableAnnotation是一个可重复用于注解一个元素的注解。
接下来,需要定义RepeatableAnnotationContainer注解类型。这基本上可称之为注解类型的容器,它必须有RepeatableAnnotation注解类型的数组。
public@interfaceRepeatableAnnotationContainer {
RepeatableAnnotation [] value();
}
现在, Repeatable 注解可多次应用于任何元素。
@RepeatableAnnotation (“I am annotating the class”)
@RepeatableAnnotation (“I am annotating the class again”)
@RepeatableAnnotation (“I am annotating the class for the third time”)
public classRepeatedAnnotationExample {
}
接下来,要检索程序中注解的值,首先要检索容器的数组。数组的每个元素将包含一个值。如:
@RepeatableAnnotation (“I am annotating the class”)
@RepeatableAnnotation (“I am annotating the classagain”)
@RepeatableAnnotation(“I am annotating the classfor the third time”)
publicclassRepeatableAnnotationExample {
publicstaticvoid main(String [] args) {
Class object = RepeatableAnnotationExample.class
Annotation[] annotations = object.getAnnotations();
for (Annotation annotation : annotations) {
RepeatableAnnotationContainer rac = (RepeatableAnnotationContainer) annotation;
RepeatableAnnotation [] raArray = rac.value();
for (RepeatableAnnotation ra : raArray) {
System.out.println(ra.value);
}
}
}
}
当执行上述代码时,输出为:
I am annotating the class
Iamannotatingtheclassagain
Iamannotatingtheclassfor the third time.
类型注解
Java8发布之后,注解可以应用于任何类型的使用。这意味着可在任何使用类型的地方使用注解。例如,当使用新的操作符、类型转换创建类实例时,当使用执行子句、抛出子句等实现接口时,这种形式的注解称为类型注解。
这种类型注解的目的是支持改进的Java程序分析,并确保更强的类型检查。在Java 8发布之前,Java不包含类型检查框架,但是可使用类型注解在Java程序中编写和使用类型检查框架。
例如,假设我们希望在整个程序中都不将某个特定变量赋值为null。我们可以编写一个自定义插件NonNull来检查这个并使用自定义注解注解那个特定的变量。变量声明应该是:
@NonNullString notNullString;
在编译代码时,编译器会检查潜在的问题,并在可能为变量赋空值的地方发现任何此类代码时发出警告。
自定义注解
Java允许程序员定义和实现自定义注解。定义自定义注解的语法如下:
public@interfaceCustomAnnotation { }
这将创建一个名为CustomAnnotation的新注解类型。@interface关键字用于定义自定义注解。
在定义自定义注解时,必须为该注解定义两个强制属性。尽管这里还可以定义其他属性,但这两个属性最为重要,而且是必需的。这两个属性分别是Retention Policy 和 Target.。
这两个属性以自定义注解的形式声明。另外,可以在定义自定义注解时定义注解属性。如:
@Retention (RetentionPolicy.RUNTIME)
@Target (ElementType.ELEMENT)
public@interfaceCustomAnnotation {
publicString name() default “Mr Bean”;
publicString dateOfBirth();
}
上述自定义注解中,RetentionPolicy是RUNTIME,这意味着它在运行时对JVM可用,目标是ELEMENT,且可被注解为任何元素类型。
另外,它还有两个属性:一个是带有缺省值的MrBean,另一个是没有缺省值的dateOfBirth。
注意,声明为方法的属性没有任何参数和抛出子句。此外,返回类型仅限于所述返回类型的字符串、类、枚举、注解和数组。
现在,可通过以下方式使用自定义注解:
@CustomAnnotation (dateOfBirth = “1980-06-25”)
public class CustomAnnotatedClass {
}
类似地,可以使用@Target (ElementType.METHOD)注解创建方法的自定义注解,并可用于注解任何方法。
检索注解及其属性
Java Reflection API包含几个方法,可用于在运行时从类、方法和其他元素中检索注解。包含所有这些方法的接口是 AnnotatedElement。最重要的是:
- getAnnotations(): 返回给定元素的所有注解,以及元素定义中未显式定义的注解。
- isAnnotationPresent(annotation):检查传递的注解在当前元素中是否可用。
- getAnnotation(class): 检索作为参数传递的特定注解。如果给定元素没有此注解,则返回null。
该类由java. lang .Class,java.lang.reflect.Method,和java.lang.reflect.Field,实现。
因此,它基本上可以与任何类型的Java元素一起使用。
下面的程序演示了获得已定义的自定义注解的信息方法:
publicstaticvoidmain(String [] args) {
Class object = CustomAnnotatedClass.class;
// Retrieve all annotations from the class
Annotation[] annotations = object.getAnnotations();
for( Annotation annotation : annotations ) {
System.out.println(annotation);
}
// Checks if an annotation is present
if( object.isAnnotationPresent( CustomAnnotationClass.class ) ) {
// Gets the desired annotation
Annotation annotation = object.getAnnotation(CustomAnnotationClass.class) ;
System.out.println(annotation);
}
// fetch the attributes of the annotation
for(Annotation annotation : annotations) {
System.out.println(“name: “ + annotation.name());
System.out.println(“Date of Birth: “+ annotation.dateOfBirth());
}
// the same for all methods of the class
for( Method method : object.getDeclaredMethods() ) {
if( method.isAnnotationPresent( CustomAnnotationMethod.class ) ) {
Annotation annotation = method.getAnnotation(CustomAnnotationMethod.class );
System.out.println( annotation );
}
}
}
结论
现如今,在使用J2EE堆栈来开发企业应用程序时,注解已逐渐成为这一过程中至关重要的一部分。现在,几乎所有常见的代码库都使用注解来实现不同目的,如代码质量分析、单元测试、XML解析、依赖注入等。此外,还有一些常用代码库也广泛使用到了注解,包括 Junit 、Hibernate、Spring MVC、Findbugs、JAXB和Junit。

留言点赞关注
我们一起分享AI学习与发展的干货
如转载,请后台留言,遵守转载规范