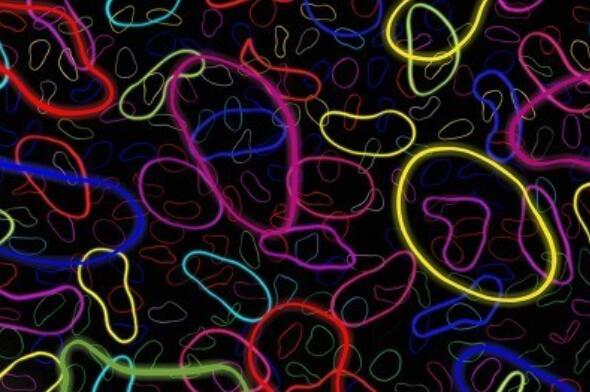
小伙伴们都知道 Java 实现多线程有两种方式:
1、继承Thread类,重写run()方法;
2、实现Runnable接口,实现run()方法
实际上还有两种方式:
3、实现Callable接口,实现call()方法;
4、通过 线程池 创建线程
接下来一个一个说明:
1、继承Thread类,重写run()方法
线程类:
package com.test.thread;
public class Thread1 extends Thread{
@Override
public void run() {
//编写业务代码
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("我是一个线程,继承Thread类,名字是:"+Thread.currentThread().getName());
}
}
测试类:
package com.test.thread;
public class Test1 {
public static void main(String[] args) {
Thread t = new Thread1();
t.start();
System.out.println("我是主线程!");
}
}
运行结果:
我是主线程!
我是一个线程,继承Thread类,名字是:Thread-0
2、实现Runnable接口,实现run()方法
线程类:
package com.test.thread;
public class Thread2 implements Runnable{
@Override
public void run() {
//编写业务代码
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("我是一个线程,实现Runnable接口,名字是:"+Thread.currentThread().getName());
}
}
测试类:
package com.test.thread;
public class Test2 {
public static void main(String[] args) {
Thread t = new Thread(new Thread2());
t.start();
System.out.println("我是主线程!");
}
}
运行结果:
我是主线程!
我是一个线程,实现Runnable接口,名字是:Thread-0
3、实现Callable接口,实现call()方法
线程类:
package com.test.thread;
import java.util.concurrent.Callable;
public class Thread3 implements Callable<String>{
@Override
public String call() throws Exception {
//编写业务代码
Thread.sleep(10000);
System.out.println("我是一个线程,实现Callable接口,名字是:"+Thread.currentThread().getName());
return "ok";
}
}
测试类:
package com.test.thread;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
public class Test3 {
public static void main(String[] args) throws InterruptedException, ExecutionException {
Callable<String> t3 = new Thread3();
FutureTask<String> task = new FutureTask<>(t3);
new Thread(task).start();
System.out.println("子线程返回值:"+task.get());
System.out.println("我是主线程!");
}
}
运行结果:
我是一个线程,实现Callable接口,名字是:Thread-0
子线程返回值:ok
我是主线程!
注:task.get()功能是获取子线程的返回值,在得到返回值之前会一直阻塞主线程main。从运行结果可以看到,“我是主线程!”在最后才输出,原因就是子线程阻塞的。
比较Callable和Runnable:
a、Callable需要实现的方法是call(),而Runnable是run()
b、Callable有返回值,而Runnable没有返回值
c、call()方法可以抛出异常,而run()方法是不能抛出异常,只能try catch捕获
4、通过线程池创建线程
线程类:
package com.test.thread;
import java.util.concurrent.Callable;
public class Thread4 implements Callable<String>{
private String no;
public Thread4(String no) {
this.no = no;
}
@Override
public String call() throws Exception {
//编写业务代码
Thread.sleep(10000);
System.out.println("我是一个线程,编号为:"+no+",实现Callable接口,名字是:"+Thread.currentThread().getName());
return no+"ok";
}
}
测试类:
package com.test.thread;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class Test4 {
public static void main(String[] args) throws InterruptedException, ExecutionException {
//线程数量
int count = 5;
//新建一个线程池
ExecutorService pool = Executors.newFixedThreadPool(count);
//用List保存多个线程的返回值
List<Future> list = new ArrayList<Future>();
for(int i=0; i<count; i++) {
Callable<String> c = new Thread4(i+"");
Future f = pool.submit(c);
list.add(f);
}
//关闭线程池,该方法调用后不会立即执行,而是等到线程执行结束再关闭线程池;
//pool.shutdownNow()这个方法会立即关闭线程池,在此处调用该方法会导致子线程异常
//因为此处子线程还在运行,关闭线程池会导致中断异常
pool.shutdown();
for(Future f: list) {
System.out.println("子线程返回值:"+f.get());
}
//可在此处调用shutdownNow,此时子线程都有返回值了,call方法执行结束了
//在此处调用该方法不会导致子线程异常
//pool.shutdownNow();
System.out.println("我是主线程!");
}
}
运行结果:
我是一个线程,编号为:0,实现Callable接口,名字是:pool-1-thread-1
我是一个线程,编号为:4,实现Callable接口,名字是:pool-1-thread-5
我是一个线程,编号为:3,实现Callable接口,名字是:pool-1-thread-4
我是一个线程,编号为:1,实现Callable接口,名字是:pool-1-thread-2
我是一个线程,编号为:2,实现Callable接口,名字是:pool-1-thread-3
子线程返回值:0ok
子线程返回值:1ok
子线程返回值:2ok
子线程返回值:3ok
子线程返回值:4ok
我是主线程!
以后面试官再问,就不要说两种了,说四种!没准面试官从茫茫人海中一眼就相中你了,O(∩_∩)O哈哈~~
欢迎朋友们关注转发点赞,谢谢~~
