分享收藏,四个PHP实现的小功能,了解一下
实例1:php浏览历史记录的方法。具体实现方法如下:
/**
* 商品历史浏览记录
* $data 商品记录信息
*/
private function _history($data)
{
if(!$data || !is_array($data))
{
return false;
}
//判断cookie类里面是否有浏览记录
if($this->_request->getCookie(‘history’))
{
$history = unserialize($this->_request->getCookie(‘history’));
array_unshift($history, $data); //在浏览记录顶部加入
/* 去除重复记录 */
$rows = array();
foreach ($history as $v)
{
if(in_array($v, $rows))
{
continue;
}
$rows[] = $v;
}
/* 如果记录数量多余5则去除 */
while (count($rows) > 5)
{
array_pop($rows); //弹出
}
setcookie(‘history’,serialize($rows),time()+3600*24*30,’/’);
}
else
{
$history = serialize(array($data));
setcookie(‘history’,$history,time()+3600*24*30,’/’);
}
}
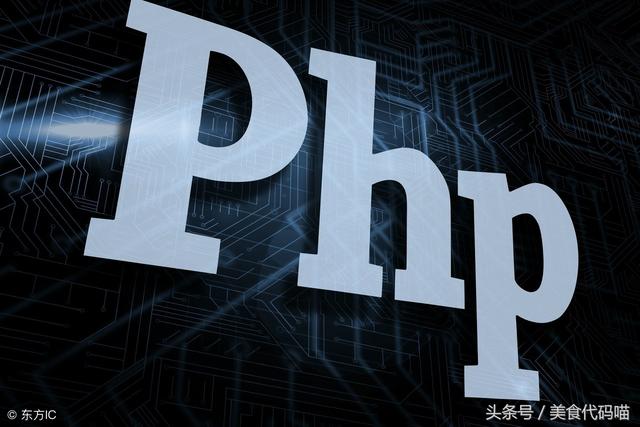
实例2:PHP+Mysql基于事务处理实现转账功能的方法,具体如下:
<?php
header(“Content-Type:text/html;charset=utf-8”);
$mysqli=new mysqli(“localhost”,”root”,””,”test”);
if(mysqli_connect_errno())
{
printf(“连接失败:%s<br>”,mysqli_connect_error());
exit();
}
$success=TRUE;
$price=8000;
$result=$mysqli->query(“select cash from account where name=’userA'”);
while($row=$result->fetch_assoc())
{
$value=$row[“cash”];
echo $value;
}
$mysqli->autocommit(0);
if($value>=$price){
$result=$mysqli->query(“UPDATE account set cash=cash-$price where name=’userA'”);
}else {
echo ‘余额不足’;
exit();
}
if(!$result or $mysqli->affected_rows!=1)
{
$success=FALSE;
}
$result=$mysqli->query(“UPDATE account set cash=cash+$price where name=’userB'”);
if(!result or $mysqli->affected_rows!=1){
$success=FALSE;
}
if($success)
{
$mysqli->commit();
echo ‘转账成功!’;
}else
{
$mysqli->rollback();
echo “转账失败!”;
}
$mysqli->autocommit(1);
$query=”select cash from account where name=?”;
$stmt=$mysqli->prepare($query);
$stmt->bind_param(‘s’,$name);
$name=’userA’;
$stmt->execute();
$stmt->store_result();
$stmt->bind_result($cash);
while($stmt->fetch())
echo “用户userA的值为:”.$cash;
$mysqli->close();
?>
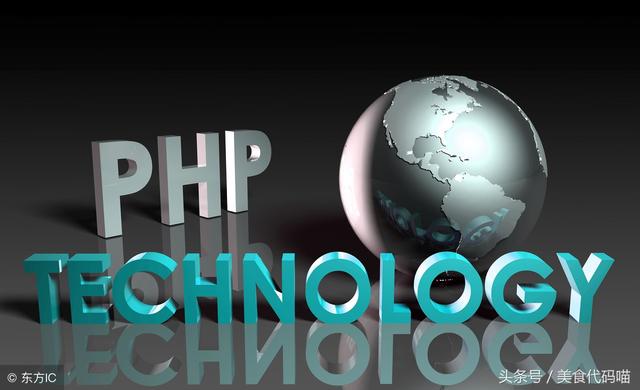
实例3:php实现html标签闭合检测与修复方法,具体如下:html标签闭合检测与修复,说的有点大 , 并没有考虑的很完整,没有使用正则表达式, 适用于html文件中只有开始标签没有结束标签, 是有结束标签没有开始标签的情况。标签闭合的位置需要根据需求调整
<?php
$str = ‘
<p data=”<li></li>”>
<img src=””/>
<p2>
<a>content</a>
</p2>
<ul>
<li>
</li>
</ul>
<p>
content full
</p>
this is content</test1>
this is content</test2>
<test4 data=”liujinjing”> This is cont
<li></li>
<test3 data=”liujinjing”> This is content
<p3>
</p3>
</p4>
</p>
</p>
<p6 style=”width:90px; “> this is content’;
$str_len = strlen ($str);
//记录起始标签
$pre_data = array();
//记录起始标签位置
$pre_pos = array();
$last_data = array();
$error_data = array();
$error_pos = array();
$i = 0;
//标记为 < 开始
$start_flag = false;
while( $i < $str_len ) {
if($str[$i]==”<” && $str[$i+1]!=’/’ && $str[$i+1]!=’!’) {
$i++;
$_tmp_str = ”;
//标记为 < 开始
$start_flag = true;
//标记空白
$space_flag = false;
while($str[$i]!=”>” && $str[$i]!=”‘” && $str[$i]!='”‘ && $str[$i] !=’/’ && $i<$str_len){
if($str[$i]==’ ‘) {
$space_flag = true;
}
if(!$space_flag) {
$_tmp_str .= $str[$i];
}
$i++;
}
$pre_data[] = $_tmp_str;
$pre_pos[] = $i;
} else if ($str[$i]==”<” && $str[$i+1]==’/’) {
$i += 2;
$_tmp_str = ”;
while($str[$i]!=”>” && $i<$str_len){
$_tmp_str .= $str[$i];
$i++;
}
$last_data[] = $_tmp_str;
//查看开始标签的上一个值
if(count($pre_data)>0) {
$last_pre_node = getLastNode($pre_data, 1);
if($last_pre_node == $_tmp_str) {
//配对上, 删除对应位置的值
array_pop($pre_data);
array_pop($pre_pos);
array_pop($last_data);
} else {
//没有配对上, 有两种情况
//情况一: 只有闭合标签, 没有开始标签
//情况二:只有开始标签, 没有闭合标签
array_pop($last_data);
$error_data[] = $_tmp_str;
$error_pos[] = $i;
}
} else {
array_pop($last_data);
$error_data[] = $_tmp_str;
$error_pos[] = $i;
}
}else if ($str[$i]==”<” && $str[$i+1]==”!”) {
$i++;
while($i<$str_len) {
if($str[$i]==”-” && $str[$i+1]==”-” && $str[$i+2]==”>”) {
$i++;
break ;
} else {
$i++;
}
}
$i++;
}else if($str[$i]==’/’ && $str[$i+1]==’>’) {
//跳过自动单个闭合标签
if($start_flag) {
array_pop($pre_data);
array_pop($pre_pos);
$i+=2;
}
}else if($str[$i]==”/” && $str[$i+1]==”*”){
$i++;
while($i<$str_len) {
if($str[$i]==”*” && $str[$i+1]==”/”) {
$i++;
break;
} else {
$i++;
}
$i++;
}
}else if($str[$i]==”‘”){
$i++;
while($str[$i]!=”‘” && $i<$str_len) {
$i++;
}
$i++;
} else if($str[$i]=='”‘){
$i++;
while($str[$i]!='”‘ && $i<$str_len ) {
$i++;
}
$i++;
} else {
$i++;
}
}
//确定起始标签的位置
function confirm_pre_pos($str, $pre_pos){
$str_len = strlen($str);
$j=$pre_pos;
while($j < $str_len) {
if($str[$j] == ‘”‘) {
$j++;
while ($j<$str_len) {
if($str[$j]=='”‘) {
$j++;
break;
}
$j++;
}
}
else if($str[$j] == “‘”) {
$j++;
while ($j<$str_len) {
if($str[$j]==”‘”) {
$j++;
break;
}
$j++;
}
}
else if($str[$j]==”>”) {
$j++;
while ($j<$str_len) {
if($str[$j]==”<“) {
//退回到原有内容位置
$j–;
break;
}
$j++;
}
break;
}
else {
$j++;
}
}
return $j;
}
//确定起始标签的位置
function confirm_err_pos($str, $err_pos){
$j=$err_pos;
$j–;
while($j > 0) {
if($str[$j] == ‘”‘) {
$j–;
while ($j<$str_len) {
if($str[$j]=='”‘) {
$j–;
break;
}
$j–;
}
}
else if($str[$j] == “‘”) {
$j–;
while ($j<$str_len) {
if($str[$j]==”‘”) {
$j–;
break;
}
$j–;
}
}
else if($str[$j]==”>”) {
$j++;
break;
}
else {
$j–;
}
}
return $j;
}
//获取数组的倒数第num个值
function getLastNode(array $arr, $num){
$len = count($arr);
if($len > $num) {
return $arr[$len-$num];
} else {
return $arr[0];
}
}
//整理数据, 主要是向后看, 进一步进行检查
function sort_data(&$pre_data, &$pre_pos, &$error_data, &$error_pos){
$rem_key_array = array();
$rem_i_array = array();
//获取需要删除的值
foreach($error_data as $key=>$value){
$count = count($pre_data);
for($i=($count-1) ; $i>=0; $i–) {
if($pre_data[$i] == $value && !in_array($i, $rem_i_array)) {
$rem_key_array[] = $key;
$rem_i_array[] = $i;
break;
}
}
}
//删除起始标签相应的值
foreach($rem_key_array as $_item) {
unset($error_pos[$_item]);
unset($error_data[$_item]);
}
//删除结束标签相应的值
foreach($rem_i_array as $_item) {
unset($pre_data[$_item]);
unset($pre_pos[$_item]);
}
}
//整理数据, 闭合标签
function modify_data($str, $pre_data, $pre_pos, $error_data, $error_pos){
$move_log = array();
//只有闭合标签的数据
foreach ($error_data as $key => $value) {
// code…
$_tmp_move_count = 0;
foreach ($move_log as $pos_key => $move_value) {
// code…
if($error_pos[$key]>=$pos_key) {
$_tmp_move_count += $move_value;
}
}
$data = insert_data($str, $value, $error_pos[$key]+$_tmp_move_count, false);
$str = $data[‘str’];
$move_log[$data[‘pos’]] = $data[‘move_count’];
}
//只有起始标签的数据
foreach ($pre_data as $key => $value) {
// code…
$_tmp_move_count = 0;
foreach ($move_log as $pos_key => $move_value) {
// code…
if($pre_pos[$key]>=$pos_key) {
$_tmp_move_count += $move_value;
}
}
$data = insert_data($str, $value, $pre_pos[$key]+$_tmp_move_count, true);
$str = $data[‘str’];
$move_log[$data[‘pos’]] = $data[‘move_count’];
}
return $str;
}
//插入数据, $type 表示插入数据的方式
function insert_data($str, $insert_data, $pos, $type) {
$len = strlen($str);
//起始标签类型
if($type==true) {
$move_count = strlen($insert_data)+3;
$pos = confirm_pre_pos($str, $pos);
$pre_str = substr($str, 0, $pos);
$end_str = substr($str, $pos);
$mid_str = “</” . $insert_data . “>”;
//闭合标签类型
} else {
$pos = confirm_err_pos($str, $pos);
$move_count = strlen($insert_data) + 2;
$pre_str = substr($str, 0, $pos);
$end_str = substr($str, $pos);
$mid_str = “<” . $insert_data . “>”;
}
$str = $pre_str.$mid_str.$end_str;
return array(‘str’=>$str, ‘pos’=>$pos, ‘move_count’=>$move_count);
}
sort_data($pre_data, $pre_pos, $error_data, $error_pos);
$new_str = modify_data($str, $pre_data, $pre_pos, $error_data, $error_pos);
echo $new_str;
// print_r($pre_data);
// print_r($pre_pos);
// print_r($error_data);
// print_r($error_pos);
// echo strlen($str);
// foreach($pre_pos as $value){
// $value = confirm_pre_pos($str, $value);
// for($i=$value-5; $i<=$value; $i++) {
// echo $str[$i];
// }
// echo “\n”;
// }
// foreach($error_pos as $value){
// for($i=$value-5; $i<=$value; $i++) {
// echo $str[$i];
// }
// echo “\n”;
// }
?>
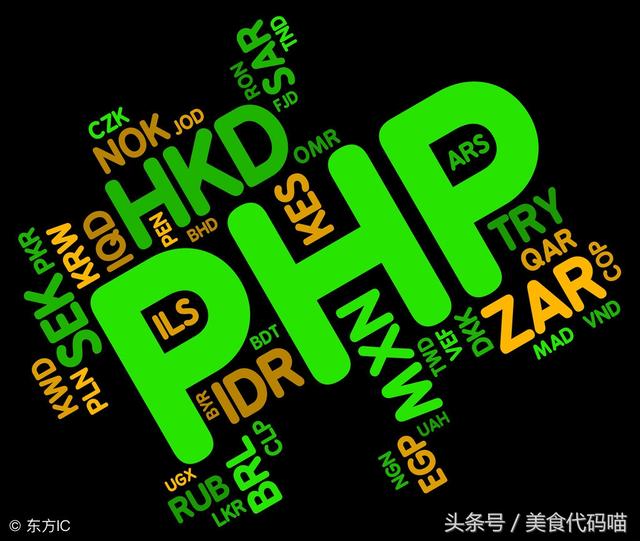
实例4:PHP实现图片自动清理的方法,具体实现方法如下:
<?php
/**
* 图片清理计划程序,删除文件下两周没有访问的文件
*/
$sRootPath = dirname(__FILE__);
//define(TIME_LINE ,”-7 day”);
//删除几天没有访问图片的时间
$dir = $sRootPath .DIRECTORY_SEPARATOR.’upload’;
$iTimeLine = strtotime(“-7 day”);
//$iTimeLine = time();
$sHandDate = date(“Ymd”);
$sLogDir = dirname(__FILE__).DIRECTORY_SEPARATOR.’Imglog’;
$sLog = $sLogDir.DIRECTORY_SEPARATOR.$sHandDate.’.txt’;
if(!file_exists($sLogDir)) mkdir($sLogDir, 0777,true);
_clearFile($dir , $iTimeLine, $sLog);
$sEnd = ‘AT’.”\\t” .date(“Y-m-d H:i:s”).”\\t”.’EXEC OVER’.”\\n”;
echo $sEnd;
error_log($sEnd, 3, $sLog);
/**
* 清除文件操作,传入需要清除文件的路径
* @param unknown_type $sPath
*/
function _clearFile($sPath, $iTimeLine, $sLog){
if(is_dir($sPath)){
$fp = opendir($sPath);
while(!false == ($fn = readdir($fp))){
if($fn == ‘.’ || $fn ==’..’) continue;
$sFilePath = $sPath.DIRECTORY_SEPARATOR.$fn;
_clearFile($sFilePath ,$iTimeLine, $sLog);
}
}else{
if($sPath != ‘.’ && $sPath != ‘..’){
//. ..文件直接跳过,不处理
$iLastView = fileatime($sPath);
if($iLastView < $iTimeLine){
if(@unlink($sPath) === true){
//echo date(“Y-m-d H:i:s”).’成功删除文件’.$sPath;
//file_put_contents($sLog,’success del file :’.$sPath.”\\n”, FILE_APPEND);
//exit;
$str =date(“Y-m-d H:i:s”).”\\t”.’success del file :’.'[‘.$sPath.’]’.”\\n”;
error_log($str, 3, $sLog);
//exit;
}
}
}
}
}
?>